Python Online Test
For jobseekers
Practice your skills and earn a certificate of achievement when you score in the top 25%.
Take a Practice TestFor companies
Test candidates with real-world problems and interview the best ones.
Sign Up to Offer this TestAbout the test
The Python online test assesses knowledge of programming in the Python language and commonly used parts of the Python Standard Library. This test requires solving live coding problems in Python.
The assessment includes work-sample tasks such as:
- Working with Python collections and language constructs.
- Using proper algorithms and data structures to optimize application performance.
- Serializing to/from JSON to handle web requests.
A good Python developer needs a solid understanding of the Python programming language and knowledge of its data structures and language constructs to write robust and maintainable code.
Sample public questions
Your company is analyzing malware that targets numerical record files stored in an array.
The malware adjusts values at the array extremes using a window of size 's' as shown in the video below:
Implement the simulate method so that the malware behavior is replicated for further study.
A gaming company is working on a platformer game. They need a function that will compute the character's final speed, given a map and a starting speed.
The terrain on which the game character moves forward is made from various pieces of land placed together. Implement the function calculate_final_speed which takes the initial speed of the character, and a list of degrees of inclination that represent the uneven terrain.
The speed of the character will increase or decrease proportionally to the incline of the land, as shown in the image below:
The magnitude of the angle of inclination will always be < 90°. The speed change occurs only once for each piece of land. The function should immediately return 0 as the final speed if an incline reduces the speed to 0 or below 0, which makes the character lose 1 life.
For example, the below code:
print(calculate_final_speed(60, [0, 30, 0, -45, 0]))
should print:
75
For jobseekers: get certified
Earn a free certificate by achieving top 25% on the Python test with public questions.
Take a Certification TestSample silver certificate
Sunshine Caprio
Java and SQL TestDomeCertificate
For companies: premium questions
Buy TestDome to access premium questions that can't be practiced.
Get money back if you find any premium question answered online.
Ready to interview?
Use these and other questions from our library with our
Code Interview Platform.
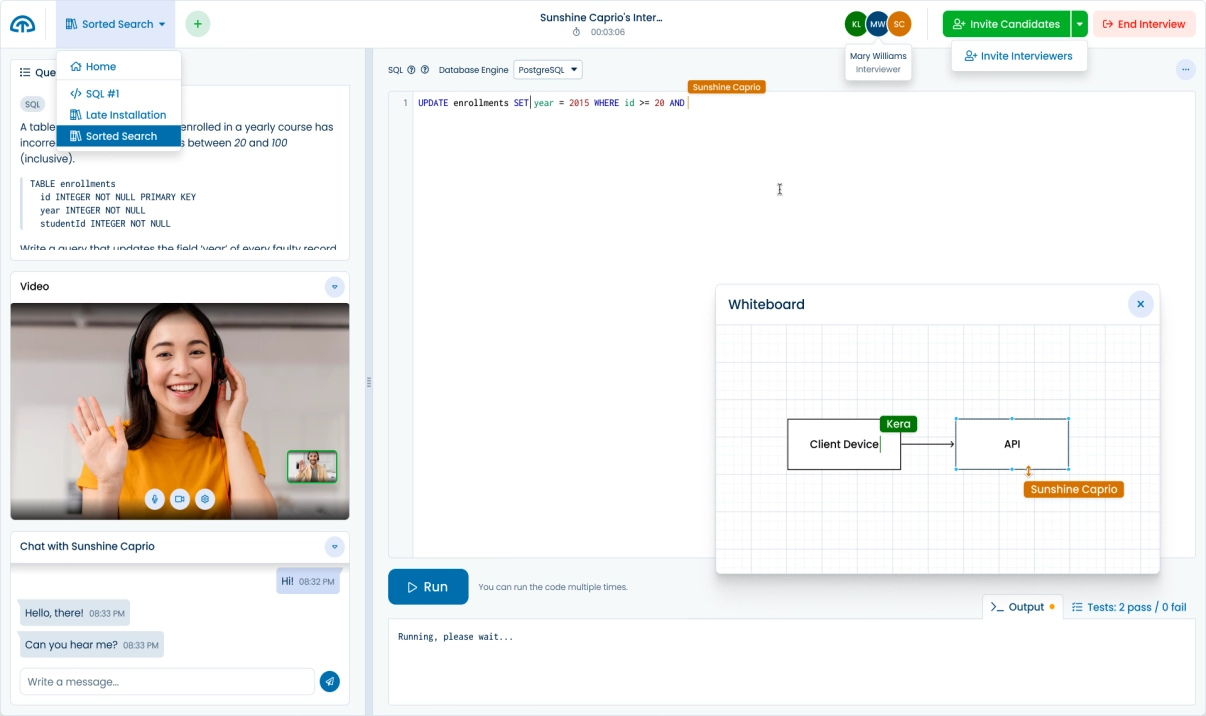
67 more premium Python questions
Category Tree, Kilometer Converter, Chain Link, Unique Numbers, Moving Total, Max Sum, Reward Points, Flight Connections, Internal Nodes, Is Alpha, Hobbies, Medical Record, Read First Line, Crop Ratio, Username, Log Parser, Veterinarian, Document Store, Segment, Language Teacher, Read Write Execute, Ceramic Store, String Occurrence, Book Sale, Numbers To Text, Log Patch, Count Hobbies, Book Authors, Friend, Car Rental, Chinese Box, Stories, Driver Exam, Worker, Vectors, Random Playlist, File Format, Action Stack, Paragraph, Cargo Ship, Tuple Slice, Greeter, Node, Students, Calories Burned, Popular Book, Paper Strip, Construction Game, Parking Allocation, Circuit Simulator, Date Transform, Planet Search, Special Actions, Company Stock, Chemical Machine, Adventure Game, Seasonal Tourism, Candies, Ecological Experiment, Jobs Time, Digital Flasks, Shipping, Flimsy Bridge, Christmas Lights, Airport Networks, Procedural Generator, Automated Forklift.
Skills and topics tested
- Python
- Dictionary
- Exceptions
- Inheritance
- Method Overriding
- OOP
- Linked List
- Algorithmic Thinking
- Set
- Graphs
- Lists
- Tree
- List Comprehension
- Strings
- Iteration
- Named Tuple
- Bug Fixing
- Integer Division
- Language
- Regex
- Serialization
- XML
- Queue
- Arithmetic
- Multithreading
- Stream
- Sorting
- Monkey Patching
- Conditions
- Performance Tuning
- Data Structures
- Dynamic Programming
- Memory Management
- Closures
- Stack
- Tuples
- Recursion
- JSON
- AI Code Review
- Conditional Statements
- Video
- Loops
- Classes
- 2D Array
- Random
For job roles
- Back-End Developer
- Python Developer
- Web Developer
Sample candidate report
Need it fast? AI-crafted tests for your job role
TestDome generates custom tests tailored to the specific skills you need for your job role.
Sign up now to try it out and see how AI can streamline your hiring process!
What others say
Simple, straight-forward technical testing
TestDome is simple, provides a reasonable (though not extensive) battery of tests to choose from, and doesn't take the candidate an inordinate amount of time. It also simulates working pressure with the time limits.
Jan Opperman, Grindrod Bank
Product reviews
Used by
Solve all your skill testing needs
150+ Pre-made tests
130+ skills
Multi-skills Test
How TestDome works
Choose a pre-made test
or create a custom test
Invite candidates via
email, URL, or your ATS
Candidates take
a test remotely
Sort candidates and
get individual reports