OOP and Design Patterns Online Test
For jobseekers
Practice your skills and earn a certificate of achievement when you score in the top 25%.
Take a Practice TestFor companies
Test candidates with real-world problems and interview the best ones.
Sign Up to Offer this TestAbout the test
The Object Oriented Programming and Design Patterns online test assesses knowledge of OOP concepts such as polymorphism, inheritance, abstraction, encapsulation, as well as software design patterns.
The assessment includes work-sample tasks, such as:
- Analyzing a set of inherited classes.
- Reading UML diagrams.
- Using inversion of control to decouple components.
A good software engineer who uses a modern OOP language needs to understand core concepts of the OOP language and how to use design patterns to write reusable solutions that other developers can understand.
Sample public questions
In a language that supports the OOP paradigm, we have the following code that serializes the content of a shopping cart to JSON format:
class ShoppingCart
private content : Dictionary<Int, String>
public function serialize() : String
return new JsonSerializer().serialize(content.clone())
end function
end class
class JsonSerializer
public function serialize(value : Dictionary<Int, String>) : String
// Code that serializes dictionary to JSON format and returns it as string
end function
end class
A client wants to allow loosely coupled plugins to be able to serialize the shopping cart content to their own formats (e.g., XML). Select lines of code that, together, would extend the code above to allow this.
Your team has started to write software for managing a vehicle storehouse using a programming language that supports OOP. This is the class hierarchy you plan to use:
class Vehicle
class Car inherits Vehicle
class Bicycle inherits Vehicle
class MountainBike inherits Bicycle
class Downhill inherits MountainBike
Objects of which type can be assigned to a variable of type Bicycle?
For jobseekers: get certified
Earn a free certificate by achieving top 25% on the OOP and Design Patterns test with public questions.
Take a Certification TestSample silver certificate
Sunshine Caprio
Java and SQL TestDomeCertificate
For companies: premium questions
Buy TestDome to access premium questions that can't be practiced.
Get money back if you find any premium question answered online.
Ready to interview?
Use these and other questions from our library with our
Code Interview Platform.
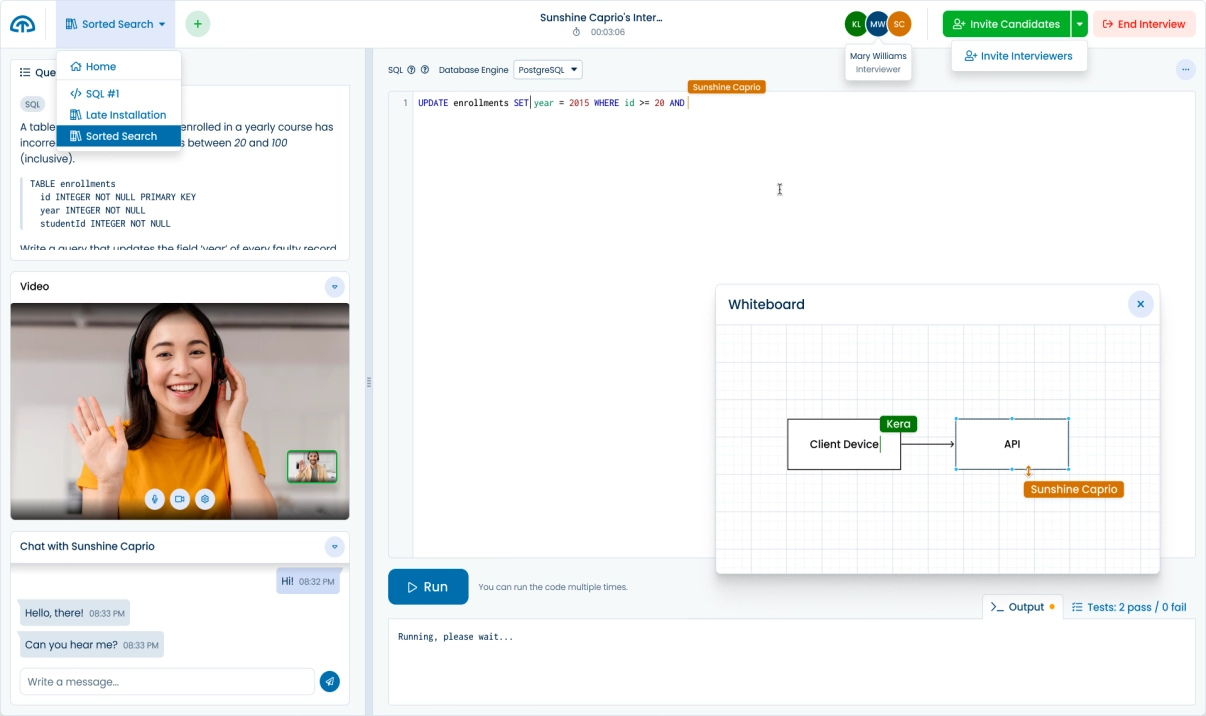
6 more premium OOP and Design Patterns questions
Panel System, Engine, UML Diagrams, Advertising Platform, Welcome Screen, Mock Configuration.
Skills and topics tested
- OOP & Design Patterns
- Behavioral Patterns
- Creational Patterns
- Design Patterns
- Inheritance
- Method Overriding
- UML
- Inversion of Control
For job roles
- Back-End Developer
- Full-Stack Developer
- Mobile Developer
- Software Developer
- Web Developer
Sample candidate report
Need it fast? AI-crafted tests for your job role
TestDome generates custom tests tailored to the specific skills you need for your job role.
Sign up now to try it out and see how AI can streamline your hiring process!
What others say
Simple, straight-forward technical testing
TestDome is simple, provides a reasonable (though not extensive) battery of tests to choose from, and doesn't take the candidate an inordinate amount of time. It also simulates working pressure with the time limits.
Jan Opperman, Grindrod Bank
Product reviews
Used by
Solve all your skill testing needs
150+ Pre-made tests
130+ skills
Multi-skills Test
How TestDome works
Choose a pre-made test
or create a custom test
Invite candidates via
email, URL, or your ATS
Candidates take
a test remotely
Sort candidates and
get individual reports